Learn Go constants — A visual guide
Go’s typed and untyped constants are two crucial concepts you need to understand.

👉 Don’t forget that there are a bunch of more information in the code examples below, so be sure to click and try them.
Why might you use constants?
You don’t want to type magical values everywhere in your code, so, declare them with constants and reuse them again.
Magical values are not safe, you may type them incorrectly, so, a constant is your safer alternative. Also, it’s more enjoyable to see a constant instead of a magic value; People will understand more easily what’s going on in your code.
We hope that we’ll get some speed benefits of using constants, because, the compiler will be able to do more optimizations, because, it knows that the value of the constant will never change.
My favorite is the untyped constants. They’re really a genius idea. You’ll get flexibility and high-precision calculations when you use untyped constants.
Typed constants
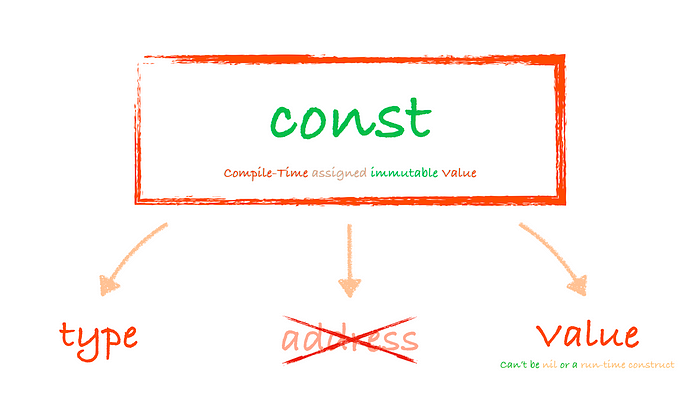
Type → Boolean, rune, numerics, or string.
Value → Assigned at its declaration at compile-time.
Address → You can’t access it from its memory address (unlike variables).
→ You can’t change its value after you declare it.
→ You can’t use run-time constructs like variables, pointers, arrays, slices, maps, structs, interfaces, function calls or function values.
Typed constant declaration
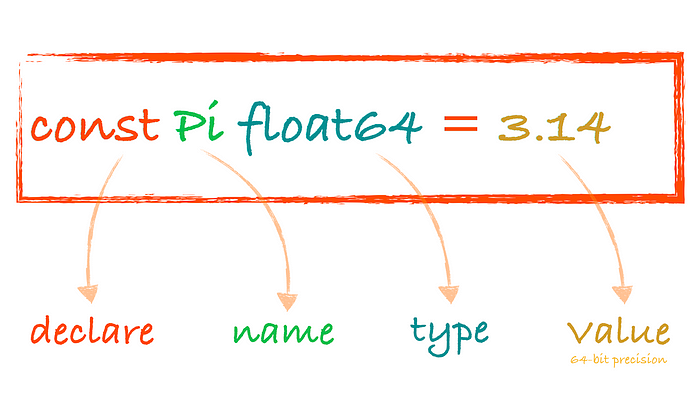
Declares a typed constant named Pi with a type of float64 and value of 3.14.
Run and try the code example, here.
Multiple constant declarations
Declares multiple constants which have different types and values in the same statement.
- When a constant’s type and value is not declared, it will get it from the previous constant. Here above, Pi2 gets its type and value from Pi.
- Age constant is declared with a new value. And, it gets its type through the assigned value of 10 (it has a default type of int).
- Multiple constants can be defined on the same line as well just as in multiple variables declaration.
Untyped constants
They have great properties like high-precision calculations and ability to use them in all of the numeric expressions without declaring a type etc. I’ll tell you about these below. They’re like the wildcards of Go.
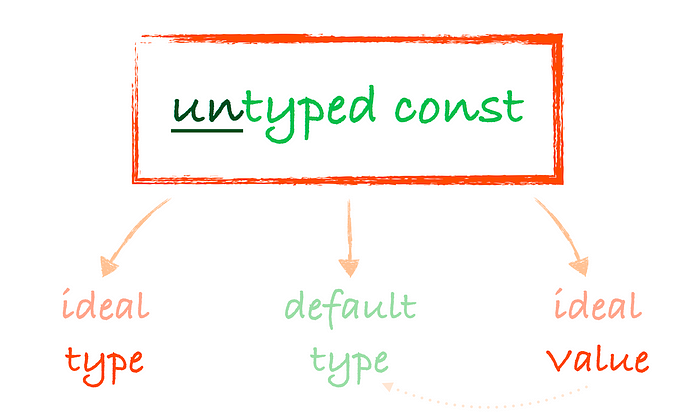
Ideal Type → A hidden type different than Go’s usual types.
Ideal Value → Lives in the ideal-values space and has a default-type.
Default Type → Depends on the ideal value.
Untyped constant declaration
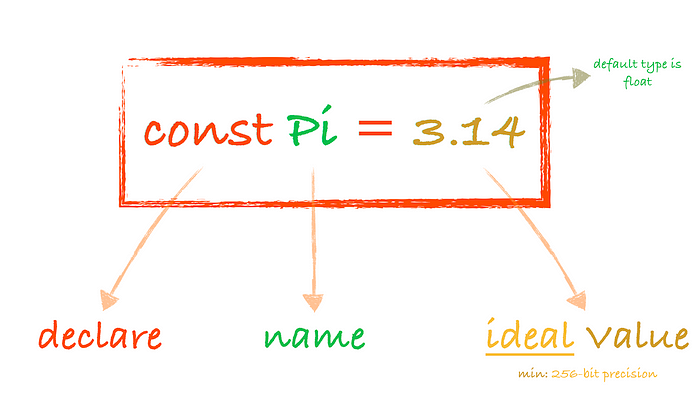
Declares an untyped constant named Pi with an ideal numeric value of 3.14 and default type of float.
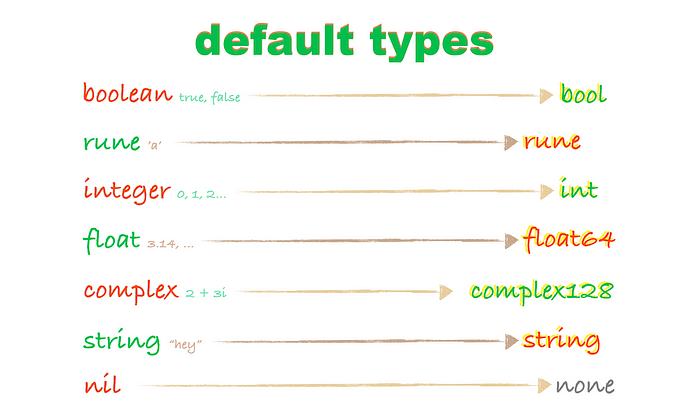
Try the code example, here.
👉 Default type will be converted to a usual type when necessary.
High-Precision calculations
If you stay in the untyped constants realm, there is no-speed-limit! But, when you use them in a variable, the speed-limit applies.
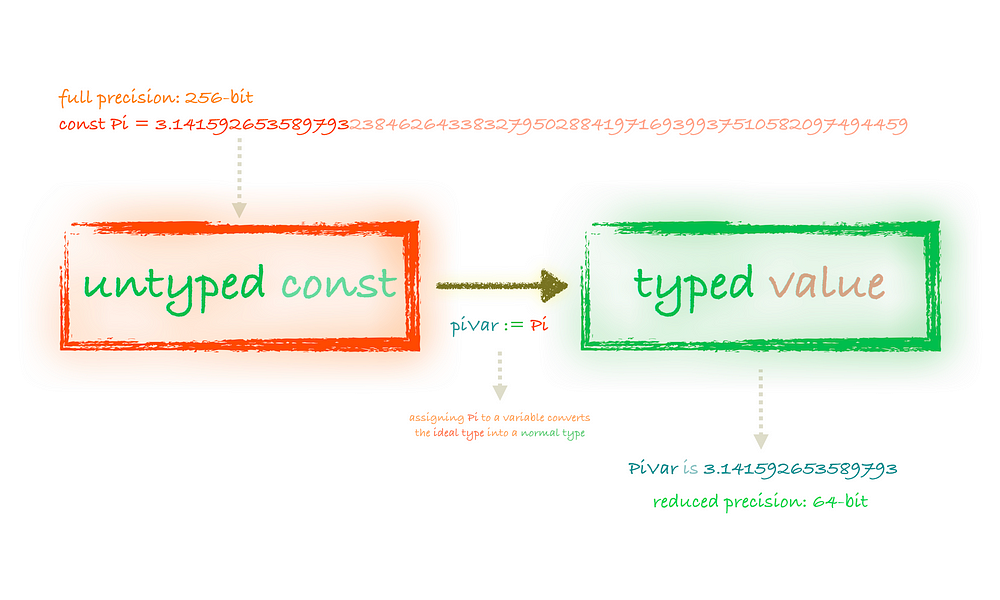
👉 When you assign it to a variable, an untyped constant’s precision is reduced and its default type is converted to Go’s normal types.
Run the code example here.
Flexible expressions
You can use untyped constants to temporarily escape from Go’s strong type system until their evaluation in a type-demanding expression.
I use them all the time in my code to get rid of declaring things unnecessarily when I don’t need a strong type. So, don’t declare a type with a constant, if you don’t really need it.
Run the code examples:
Scopes and Shadowing
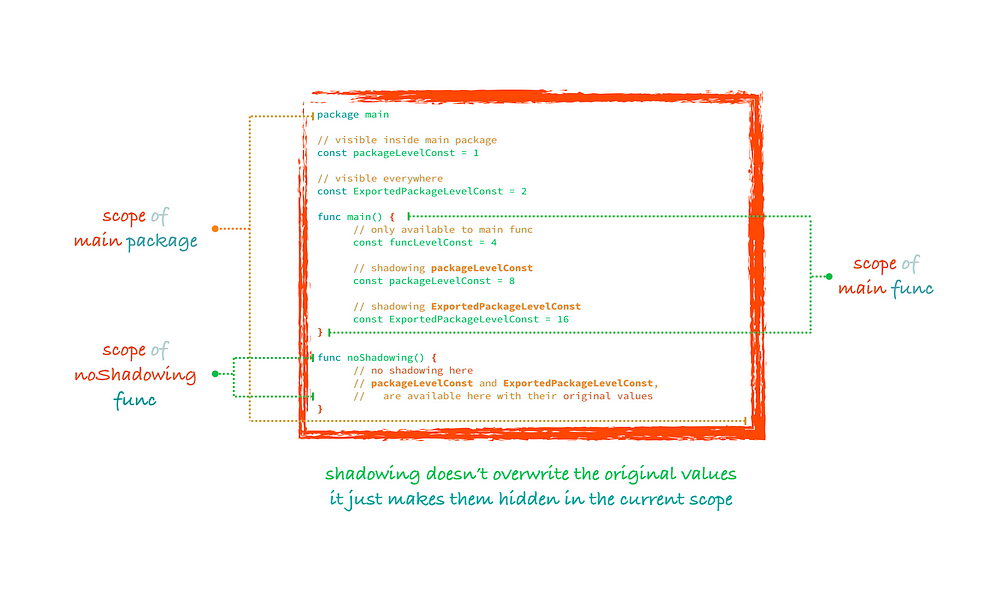
👉 A constant can only be used within its declared scope. If you redeclare a constant in an inner scope with the same name again, it’ll be a new constant that is only visible to that inner scope and it will shadow the outer scope’s constant. See the code example, here.

Alright, that’s all for now. Thank you for reading so far.
Let’s stay in touch:
- 📩 Join my newsletter
- 🐦 Follow me on twitter
- 📦 Get my Go repository for free tutorials, examples, and exercises
- 📺 Learn Go with my Go Bootcamp Course
- ❤️ Do you want to help? Please clap and share the article. Let other people also learn from this article.